I was trying to use SignalR 2 for one of my project and successfully implemented a progress bar using it.
Everything was working fine in our test server also. But fortunately i tested this SignalR 2 based progress bar in Web-garden mode of IIS,
Woww surprised nothing is working. Thanks God, because our production servers are load balanced Web Farm.
Any way found solution in Microsoft documentation. I will discuss that below.
Hope you have an understanding of SignalR.
1. Create an MVC 5 app.
2. Add Nuget package for SignalR.
Install-Package Microsoft.AspNet.SignalR
3.Now Paste the below code in your cshtml.
<input type="button" value="Submit" id="btnSubmit" />
<input type="hidden" id="connectionid" />
<div id="uploadModal"></div>
@section scripts
{
<!--Reference the SignalR library. -->
<script src="~/Scripts/jquery.signalR-2.2.0.min.js"></script>
<!--Reference the autogenerated SignalR hub script. -->
<script src="~/signalr/hubs"></script>
<script type="text/javascript">
$(document).ready(function() {
var con = $.connection.uploaderHub;
$.connection.hub.start().done(function() {
$("#connectionid").val($.connection.hub.id);
}).fail(function(e) {
alert('There was an error');
console.error(e);
});
con.client.print = function() {
$("#uploadModal").append("Vivek");
};
$("#btnSubmit").click(function() {
var connectionid = $("#connectionid").val();
var url = '@Url.Action("Test", "Home")';
url = url + '?connectionid=' + connectionid;
$.ajax({
type: "POST",
url: url,
dataType: 'json',
contentType: "application/json; charset=utf-8",
success: function(messages) {
},
error: function() {
}
});
});
});
</script>
}
4. Now create an action in your controller.
[HttpPost]
public ActionResult Test(string connectionid)
{
var hubContext = GlobalHost.ConnectionManager.GetHubContext<UploaderHub>();
hubContext.Clients.Client(connectionid).print();
return Json(new { Success = "True" });
}
5. Create a SignalR hub.
using Microsoft.AspNet.SignalR;
namespace SignalR.Controllers
{
public class UploaderHub:Hub
{
}
}
6. In your OWIN Startup.cs add the code below.
using Microsoft.Owin;
using Owin;
[assembly: OwinStartupAttribute(typeof(SignalR.Startup))]
namespace SignalR
{
public partial class Startup
{
public void Configuration(IAppBuilder app)
{
app.MapSignalR();
ConfigureAuth(app);
}
}
}
Deploy this to IIS and click submit button, Which will print the text defined in client side print method.
Now increase the worker process count in your application pool to 5, Also set processor affinity true.
Try the same after some refresh, You will found the messaging is not working.
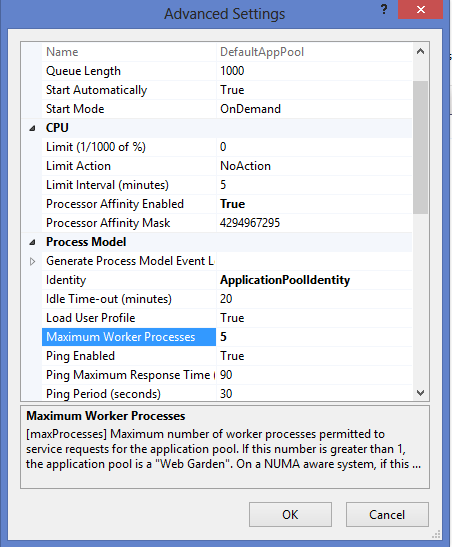
To Solve this you can use another SignalR package for SQL Server
Install-Package Microsoft.AspNet.SignalR.SqlServer -Version 2.2.0
Then update your OWIN Startup.cs add this.
using Microsoft.AspNet.SignalR;
using Microsoft.Owin;
using Owin;
[assembly: OwinStartupAttribute(typeof(SignalR.Startup))]
namespace SignalR
{
public partial class Startup
{
public void Configuration(IAppBuilder app)
{
string sqlConnectionString = "Connecton string to your SQL DB";
GlobalHost.DependencyResolver.UseSqlServer(sqlConnectionString);
app.MapSignalR();
ConfigureAuth(app);
}
}
}
Which will create some tables, in the database you specified in your connection string.
And now debug…
You must be logged in to post a comment.